How to convert array of array into set in Python 3? [duplicate]
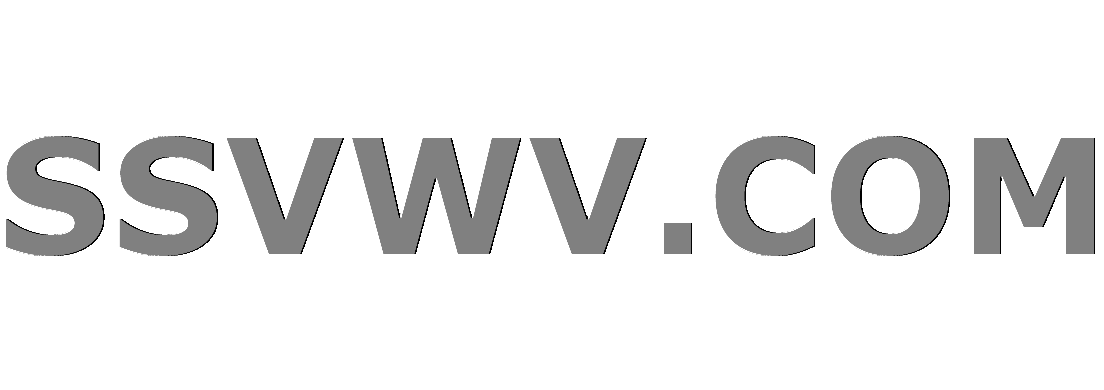
Multi tool use
Clash Royale CLAN TAG#URR8PPP
How to convert array of array into set in Python 3? [duplicate]
This question already has an answer here:
What I have as input:
list: [[2, 4], [2, 6], [2, 8]]
What I want as output:
set: 2,4,6,8
What I'm currently doing(not working):
def convert(input_list):
all_nums = set([arr[0], arr[1]] for arr in input_list)
return all_nums
I know I can manually iterate through parent array and add contents of child array to the set
like this:
set
def convert(input_list):
all_nums = set()
for inner_list in input_list:
all_nums.add(inner_list[0])
all_nums.add(inner_list[1])
return all_nums
all_nums.add(inner_list[0], inner_list[1])
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
Yup..mistake on my part. Updated the ques
– noobie
Aug 10 at 15:39
Where are your 6 and 8 coming from in your expected set? Do you just want a set of all numbers in the flattened input list? In this case
1, 2, 3, 4
?– Engineero
Aug 10 at 15:40
1, 2, 3, 4
He updated his input, his output makes sense now.
– user3483203
Aug 10 at 15:40
@user3483203 In second approach can I do something like all_nums.add(inner_list[0], inner_list[1])?
– noobie
Aug 10 at 15:47
5 Answers
5
Simply:
my_list = [[2, 4], [2, 6], [2, 8]]
my_set = e for l in my_list for e in l
This is using a "set comprehension", which is a squashed down version of:
my_list = [[2, 4], [2, 6], [2, 8]]
my_set = set()
for l in my_list:
for e in l:
my_set.add(e)
Alternatively, you could do:
my_list = [[2, 4], [2, 6], [2, 8]]
my_set = set()
for l in my_list:
my_set.update(l)
(Variable names shamelessly stolen from modesitt.)
In second approach can I do something like all_nums.add(inner_list[0], inner_list[1])?
– noobie
Aug 10 at 15:45
You need double brackets so you're adding a tuple (immutable, hashable list), but yes. Alternatively, you could do
my_set = (l[0], l[1]) for l in my_list
or my_set = tuple(l) for l in my_list
or my_set = (x, y) for x, y in my_list
.– wizzwizz4
Aug 10 at 15:48
my_set = (l[0], l[1]) for l in my_list
my_set = tuple(l) for l in my_list
my_set = (x, y) for x, y in my_list
in second approach doing
all_nums.add((inner_list[0], inner_list[1]))
gives (2, 8), (2, 6), (2, 4)
but not 2, 4, 6, 8– noobie
Aug 10 at 16:07
all_nums.add((inner_list[0], inner_list[1]))
(2, 8), (2, 6), (2, 4)
I thought that was what you wanted...
– wizzwizz4
Aug 10 at 16:18
is there a way I can get 2, 4, 6, 8 without adding them separately inside for-loop (see the second approach)?
– noobie
Aug 10 at 16:21
One approach using itertools.chain
and set
itertools.chain
set
Ex:
from itertools import chain
l = [[1, 2], [1, 3], [1, 4]]
print(set(chain.from_iterable(l)))
Output:
set([1, 2, 3, 4])
chain
You can try this:
from itertools import chain
l = [[1, 2], [1, 3], [1, 4]]
l = list(chain.from_iterable(l))
set_l = set(l)
There's no need for the intermediate list, by the way.
– wizzwizz4
Aug 10 at 15:43
I would do the following
set_ = e for l in my_list for e in l
Can I steal your variable names? There better than my
x
and y
(e
lement and l
ist, I assume yours are) but my answer has 2 upvotes.– wizzwizz4
Aug 10 at 15:43
x
y
e
l
of course! you won that race condition ;) @wizzwizz4
– modesitt
Aug 10 at 15:44
You can use set comprehension:
l = [[2, 4], [2, 6], [2, 8]]
print(i for s in l for i in s)
This outputs:
8, 2, 4, 6
Do you mean the set should be 1,2,3,4?
– Brandon Wang
Aug 10 at 15:38